Image optimization involves delivering images with the smallest possible file size while maintaining visual quality. Optimizing images means saving bytes and improving performance for your website. The fewer bytes per image, the faster the browser can download and render the content on your users’ screens.
Web developers build their web platforms using a variety of programming languages, such as PHP, Python and Ruby.
In this article, we’ll take a look at how to perform and implement image optimization in one of the most common server-side languages on the web, PHP. However, before we dive right in, let’s explore why image optimization is so important.
Why Optimize Images?
The faster images load on your website, the higher your conversion rates will be. In fact, half of your visitors expect your website to load within two seconds. Surveys from akamai.com and Gomez reveal that:
- 73 percent of mobile internet users report that they have experienced problems with page load times on their devices.
- A one-second delay in page load time can decrease visitor satisfaction by 16 percent and can lead to a drop in conversion of 7 percent or more.
- Load time is a major contributing factor to page abandonment, which increases as a percentage with every second of load time. Nearly 40 percent of users abandon a page after 3 seconds.
Optimizing Images in PHP
PHP has some built-in functions that can be used to optimize images, such as imagejpeg(). This function takes the path of the output image and quality specification ranging from 1 to 100.
Note: Ensure that the GD library for PHP is installed.
<?php function compress_image($source_url, $destination_url, $quality) { $info = getimagesize($source_url); if ($info['mime'] == 'image/jpeg') $image = imagecreatefromjpeg($source_url); elseif ($info['mime'] == 'image/gif') $image = imagecreatefromgif($source_url); elseif ($info['mime'] == 'image/png') $image = imagecreatefrompng($source_url); imagejpeg($image, $destination_url, $quality); return $destination_url; } ?>
The higher the number, the better the quality, but unfortunately the larger the size. You also can resize images with functions like imagecopyresampled and imagecopyresized.
Image Optimizer
This is a PHP library written by Piotr Sliwa for optimizing image files. Under the hood, it uses optipng, pngquant, jpegoptim and a few other libraries. With this library, your image files can be 10 percent to 70 percent smaller without losing their visual appeal.
Note: You have to ensure these other libraries like optipng, pngquant and others are installed on your server.
You will optimize an image like so:
$factory = new \ImageOptimizer\OptimizerFactory(); $optimizer = $factory->get(); $filepath = __DIR__ . '/images/prosper.png'; /* path to image */ $optimizer->optimize($filepath); //optimized file overwrites original one
You can also specify an optimizer before optimizing an image:
$optimizer = $factory->get(); //default optimizer is `smart` $pngOptimizer = $factory->get('png'); //png optimizer $jpgOptimizer = $factory->get('jpegoptim'); //jpegoptim optimizer etc.
PHP Image Cache
This is a simple PHP class that compresses images on-the-fly to reduce load time and web page resource management. It accepts an image, compresses it, then moves and caches it in the user’s browser. It then returns the new source of the image.
<?php require_once 'ImageCache.php'; $imagecache = new ImageCache(); $imagecache->cached_image_directory = dirname(__FILE__) . '/images/cached'; $cached_src = $imagecache->cache( 'images/unsplash1.jpeg' ); ?>
You also can use Composer to download it:
Imagick
Imagick is a native PHP extension to create and modify images using the ImageMagick API. One of the ways of using Imagick to optimize an image is:
<?php // Create new imagick object $im = new Imagick("File_Path/Image_Name.jpg"); // Optimize the image layers $im->optimizeImageLayers(); // Compression and quality $im->setImageCompression(Imagick::COMPRESSION_JPEG); $im->setImageCompressionQuality(25); // Write the image back $im->writeImages("File_Path/Image_Opti.jpg", true); ?>
I have given the image a quality of 25 and also set the compression type to JPEG. And the image layers have been optimized with the code above.
Now, there are so many wonderful things you can do with Imagick that I might not be able to cover. Check out this platform for more information.
Cloudinary: An Easy Optimization Alternative
Using Cloudinary, you can optimize your images, quickly and easily, regardless of the programming language you work with. Cloudinary automatically performs certain optimizations on all transformed images by default. Its integrated, fast CDN delivery also helps to get all the image resources to your users quickly.
Cloudinary offers:
Automatic quality and encoding: Using the q_auto parameter, the optimal quality compression level and optimal encoding settings are selected based on the specific image content, format and the viewing browser. The result is an image with good visual quality and a reduced file size. For example,
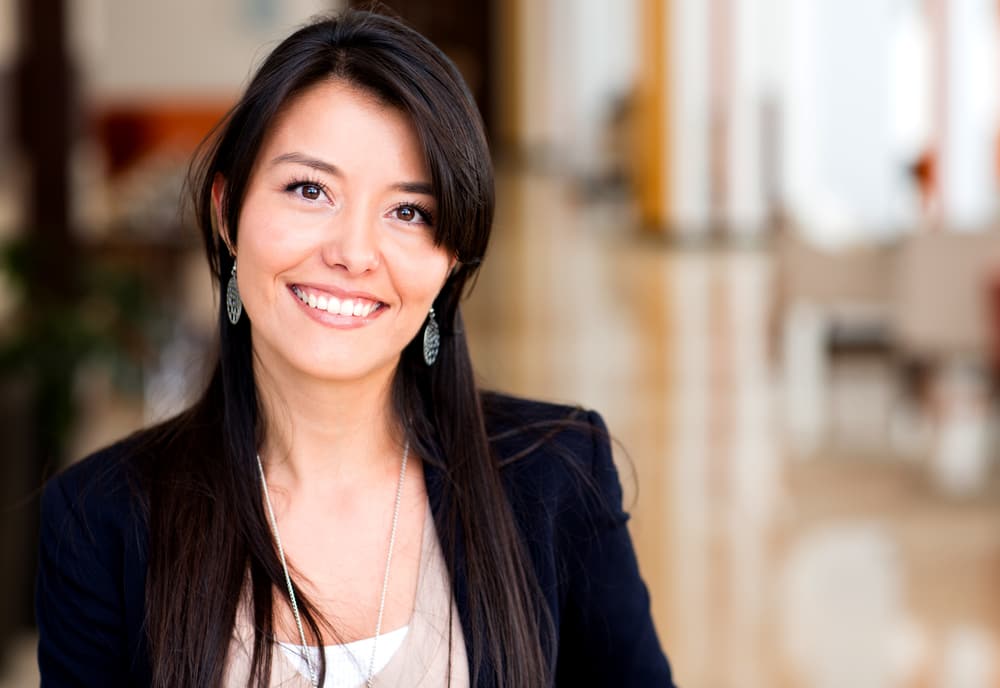
You also can use q_auto:best, q_auto:low, q_auto:good or q_auto:eco to adjust the visual quality of your images.
Automatic formatting: The f_auto parameter enables Cloudinary to analyze the image content and select the best format to deliver. For example, it can automatically deliver images as WebP to Chrome browsers or JPEG-XR to Internet Explorer browsers, while using the original format for all other browsers. Using both f_auto and q_auto, Cloudinary will still generally deliver WebP and JPEG-XR to the relevant browsers, but might deliver selected images as PNG-8 or PNG-24 if the quality algorithm determines that is optimal.
Resizing and cropping images with w and h parameters: Using the width and height parameters in URLs, you can resize the images with Cloudinary like so:
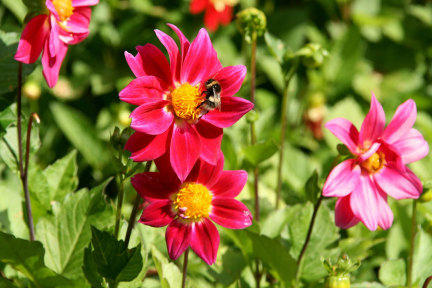
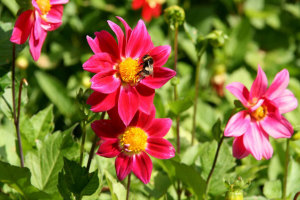
It maintains the aspect ratio, but resizes the image to whatever height and width you desire.
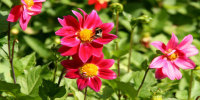
Cloudinary supports the following image cropping modes: scale, fit, mfit, fill, lfill, limit, pad, lpad, mpad, crop, thumb, imagga_crop and imagga_scale.
Conclusion
One of the best ways to increase page performance is via image optimization, so you will want to take some time to ensure that all your images are properly optimized and cached.
We have highlighted how to optimize images using PHP and Cloudinary. But these tips are just a start. Check out how to optimize your JPEG images without compromising quality for more ideas.
Cloudinary provides many options for optimizing your images. Feel free to dive in and explore them.
![]() |
Prosper Otemuyiwa is a Food Ninja, Open Source Advocate & Self-acclaimed Developer Evangelist. |